How to fix object is possibly null in TypeScript
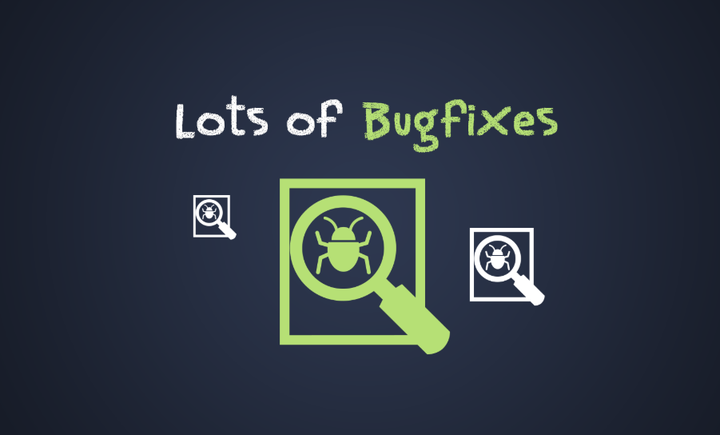
TypeScript enhances JavaScript by adding optional type-checking capabilities, which help catch errors during development and save debugging time. One common issue developers encounter is the “object is possibly null” error. This article will explain why this error occurs and provide several methods to resolve it effectively.
Why Does the “Object is Possibly Null” Error Happen?
The “object is possibly null” error, also known as TS2533, occurs when TypeScript’s strict null checks are enabled (strictNullChecks: true
). This flag ensures that properties or objects might be null
or undefined
at runtime. For example:
TypeScript复制
1 | interface IBook { |
In this case, TypeScript warns that book.author
might be null
or undefined
, and accessing name
directly could cause a runtime error.
How to Fix the “Object is Possibly Null” Error
1. Using the Optional Chaining Operator (?.)
The optional chaining operator, introduced in TypeScript 3.7, allows developers to safely access deeply nested properties by checking for null
or undefined
values. For example:
TypeScript复制
1 | let arr: number[] | undefined | null; |
If the property does not exist, the expression will return undefined
.
2. Using the Non-Null Assertion Operator (!)
The non-null assertion operator (!
) tells the TypeScript compiler that you are certain the value is not null
or undefined
. For example:
TypeScript复制
1 | const myName = 'Tim' as string | undefined; |
Use this operator with caution, as it bypasses TypeScript’s type checks and can introduce runtime errors if the value is indeed null
or undefined
.
3. Using the Nullish Coalescing Operator (??)
The nullish coalescing operator (??
) returns the right-hand operand if the left-hand operand is null
or undefined
. For example:
TypeScript复制
1 | let myName: string | undefined | null; |
This operator is useful for providing default values without triggering errors.
4. Using an If Statement
For a more traditional approach, you can use an if
statement to check if the value is not null
or undefined
. For example:
TypeScript复制
1 | const myName = 'Tim' as string | undefined; |
This method ensures that the value is valid before accessing its properties.
Final Thoughts
There are multiple ways to handle the “object is possibly null” error in TypeScript, depending on your use case and coding style. The optional chaining operator is often the most straightforward solution, but you can also use non-null assertions, nullish coalescing, or traditional if
statements.
While you can disable TypeScript’s strict null checks in tsconfig.json
, this is not recommended, as it may introduce new bugs into your code. Instead, leveraging TypeScript’s powerful type-checking features can help you write more robust and error-free code.
Related Recommendations
- BugHunter
- How to Add a Folder Recursively to a Git Repository A Comprehensive Guide
- How to Fix the “Unable to Update Local Ref” Error in Git A Step-by-Step Guide
- How to Merge a Specific File from Another Branch in Git
- How to Remove a Submodule in Git A Step-by-Step Guide
- How To Abort A Rebase In Git
- How to fix “git pre-receive hook declined”?
- Why the Git Add Command Isn’t Working Troubleshooting Guide