How to Check if a File Exists in Bash
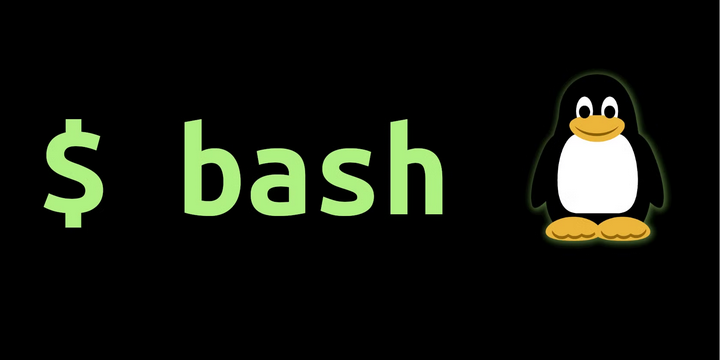
Introduction
In Bash scripting, checking if a file exists is a common task that programmers encounter daily. Whether you’re automating workflows, validating inputs, or handling errors, knowing how to perform this check efficiently is essential. This guide will walk you through the best methods to check if a file exists in Bash, complete with practical examples and tips.
Methods to Check if a File Exists in Bash
1. Using the -e
Flag
The -e
flag checks if a file exists, regardless of its type (file, directory, etc.).
1 | if [ -e "/path/to/file" ]; then |
2. Using the -f
Flag
The -f
flag checks if a file exists and is a regular file (not a directory or special file).
1 | if [ -f "/path/to/file" ]; then |
3. Using the -d
Flag
The -d
flag checks if a file exists and is a directory.
1 | if [ -d "/path/to/directory" ]; then |
4. Using the -s
Flag
The -s
flag checks if a file exists and is not empty.
1 | if [ -s "/path/to/file" ]; then |
5. Using test
Command
The test
command is an alternative to the [ ]
syntax and works the same way.
1 | if test -f "/path/to/file"; then |
Practical Example: Automating a Backup Script
Imagine you’re writing a script to back up a log file. Before copying the file, you want to ensure it exists and is not empty:
1 |
|
Tips for Using File Checks in Bash
- Quote File Paths: Always use quotes around file paths to handle spaces or special characters.
- Combine Checks: Use multiple flags together for more specific checks (e.g.,
-f
and-s
). - Error Handling: Use file checks to prevent errors in scripts, such as attempting to read a non-existent file.
- Performance: File checks are fast, but avoid unnecessary checks in loops or high-frequency scripts.
Conclusion
Checking if a file exists in Bash is a fundamental skill for programmers. By using flags like -e
, -f
, -d
, and -s
, you can ensure your scripts are robust, error-free, and efficient. Whether you’re validating inputs, automating tasks, or handling errors, these methods will help you write better Bash scripts.