Fixing "Module Not Found: React-Router-Dom" in TypeScript
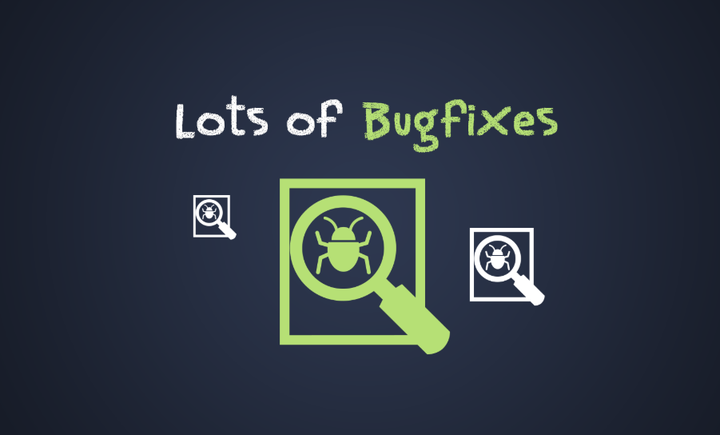
How to Fix “Module not found: Can’t resolve ‘react-router-dom’” in TypeScript with React
Introduction
When developing applications with TypeScript and React, one common issue developers face is the error: Module not found: Can’t resolve ‘react-router-dom’. This error occurs when TypeScript cannot locate the react-router-dom
module during the build process, causing the application to fail. In this article, we’ll explore the causes of this error and provide step-by-step solutions to resolve it.
Understanding the Error
The error message Module not found: Can’t resolve ‘react-router-dom’ indicates that TypeScript is unable to find the react-router-dom
module. This typically happens due to:
- Incorrect Installation: The
react-router-dom
package is not installed or installed improperly. - TypeScript Configuration Issues: The
tsconfig.json
file may lack necessary settings to recognize the module. - Project Structure Problems: Incorrect file paths or a disorganized project structure can prevent TypeScript from resolving the module.
Step-by-Step Solutions
1. Confirm Installation of react-router-dom
Ensure that react-router-dom
is installed correctly in your project. Run one of the following commands:
1 | npm install react-router-dom |
or
1 | yarn add react-router-dom |
If you’re using TypeScript, also install the type definitions:
1 | npm install -D @types/react-router-dom |
or
1 | yarn add -D @types/react-router-dom |
This ensures that both the library and its TypeScript types are available.
2. Check TypeScript Configuration
Your tsconfig.json
file should include the following settings to enable proper module resolution:
1 | { |
These options allow TypeScript to handle CommonJS modules like react-router-dom
correctly. If these settings are missing, add them and restart your TypeScript compiler.
3. Verify Project Structure
Ensure that your project structure is organized and that the react-router-dom
module is imported correctly. For example:
1 | import { BrowserRouter, Route, Routes } from 'react-router-dom'; |
If your project has a complex directory structure, consider simplifying it or using absolute paths to avoid resolution issues.
4. Clear Cache and Reinstall Dependencies
Sometimes, cached dependencies can cause issues. Clear your npm or yarn cache and reinstall all dependencies:
1 | npm cache clean --force |
or
1 | yarn cache clean |
This ensures that your project uses the latest versions of all dependencies.
5. Downgrade or Upgrade react-router-dom
(Optional)
If the issue persists, it might be due to version incompatibilities. For example, some projects work better with react-router-dom@5.2.0
instead of the latest version. To downgrade:
1 | npm install react-router-dom@5.2.0 |
Alternatively, upgrade to the latest stable version if you’re using an older one.
Conclusion
The Module not found: Can’t resolve ‘react-router-dom’ error is a common but solvable issue in TypeScript and React projects. By ensuring proper installation, configuring TypeScript correctly, organizing your project structure, and clearing cached dependencies, you can resolve this error and continue developing your application seamlessly.
For more detailed guidance, refer to the official React Router documentation
Related Recommendations
- BugHunter
- How to Add a Folder Recursively to a Git Repository A Comprehensive Guide
- How to Fix the “Unable to Update Local Ref” Error in Git A Step-by-Step Guide
- How to Merge a Specific File from Another Branch in Git
- How to Remove a Submodule in Git A Step-by-Step Guide
- How To Abort A Rebase In Git
- How to fix “git pre-receive hook declined”?
- Why the Git Add Command Isn’t Working Troubleshooting Guide