How to Run a Bash Script in Linux
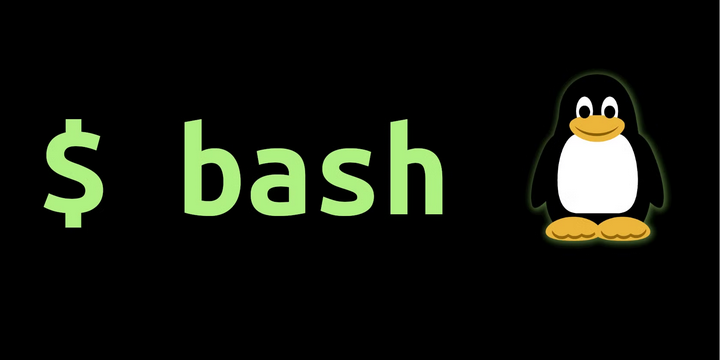
Introduction
Running Bash scripts is a fundamental skill for Linux users, especially programmers and system administrators. Whether you’re automating tasks, deploying applications, or managing servers, knowing how to execute Bash scripts efficiently is essential. This guide will walk you through the steps to run a Bash script in Linux, along with practical examples and tips.
What is a Bash Script?
A Bash script is a text file containing a series of commands that are executed by the Bash shell. It’s commonly used for automation, system administration, and repetitive tasks. Bash scripts typically have a .sh
extension, but this is not mandatory.
Steps to Run a Bash Script in Linux
1. Create a Bash Script
First, create a script file using a text editor like nano
or vim
. For example:
1 | nano myscript.sh |
Add the following code to the file:
1 |
|
Save and exit the editor.
2. Make the Script Executable
By default, script files are not executable. Use the chmod
command to add execute permissions:
1 | chmod +x myscript.sh |
3. Run the Script
There are several ways to run a Bash script in Linux:
Method 1: Using the ./
Syntax
If the script is in the current directory, use:
1 | ./myscript.sh |
Method 2: Using the bash
Command
You can explicitly call the Bash interpreter:
1 | bash myscript.sh |
Method 3: Using the Full Path
If the script is in another directory, provide the full path:
1 | /home/user/scripts/myscript.sh |
Method 4: Adding to PATH
For frequently used scripts, add the script’s directory to your PATH
environment variable:
1 | export PATH=$PATH:/home/user/scripts |
Now you can run the script from anywhere:
1 | myscript.sh |
Practical Example: Automating a Backup Task
Imagine you want to create a script that backs up a directory to a specified location:
1 |
|
Save this as backup.sh
, make it executable, and run it:
1 | chmod +x backup.sh |
Tips for Running Bash Scripts
- Shebang Line: Always include
#!/bin/bash
at the top of your script to specify the interpreter. - Permissions: Ensure the script has execute permissions using
chmod +x
. - Debugging: Use
bash -x script.sh
to debug and trace script execution. - Error Handling: Add error checks to handle unexpected issues gracefully.
- Logging: Redirect output to a log file for troubleshooting:
1
./myscript.sh > script.log 2>&1
Conclusion
Running Bash scripts in Linux is a powerful way to automate tasks and improve productivity. By following the steps outlined in this guide, you can create, execute, and manage Bash scripts with ease. Whether you’re a beginner or an experienced programmer, mastering Bash scripting is a valuable skill for Linux environments.