Mastering Git Conflict Resolution with "Ours" and "Theirs" Strategies
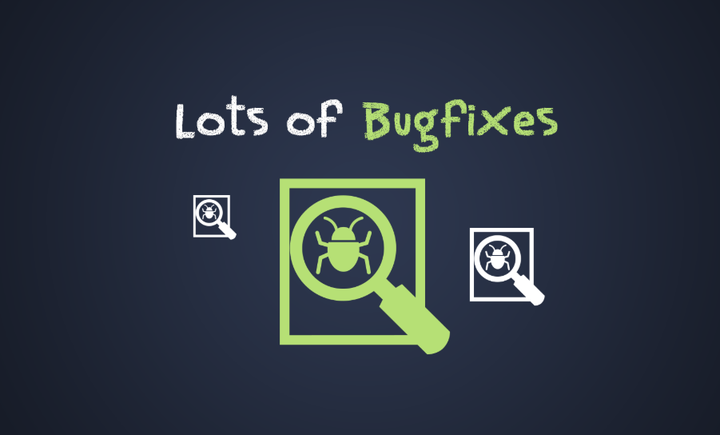
Git branching is a powerful feature that allows developers to work independently without interfering with each other’s progress. However, when it comes time to merge branches back into the main branch (e.g., main
or master
), conflicts can arise. These conflicts occur when Git is unsure how to reconcile changes between branches. Manually resolving conflicts can be time-consuming, but Git provides efficient strategies to streamline this process using the -Xours
and -Xtheirs
options.
In this article, we’ll explore the reasons behind merge conflicts and demonstrate how to resolve them quickly using these strategies. If you’re already familiar with Git internals, feel free to skip to the command examples.
Why Do Merge Conflicts Occur?
A branch in Git is a lightweight, movable pointer to a specific commit. When you create a new branch from master
using git branch feature
, a new pointer is created. As you work on your feature
branch, the master
branch may continue to evolve, especially if other branches are merged into it. This divergence can lead to conflicts when you try to merge your feature
branch back into master
.
For example, consider the following scenario:
复制
1 | E---F---G feature |
When you attempt to merge feature
into master
using git checkout master
followed by git merge feature
, Git tries to replay the changes made on feature
since it diverged from master
. If both branches modified the same file, a conflict occurs because Git doesn’t know which changes to keep.
You can abort the merge process using git merge --abort
if conflicts arise. However, resolving conflicts efficiently is often more desirable.
Resolving Conflicts with -Xtheirs
and -Xours
When you need to override changes from one branch with another, Git provides two merge strategy options: -Xtheirs
and -Xours
. These options allow you to resolve conflicts quickly without manual intervention.
1. Overriding Changes in master
with feature
Branch
If you want to override changes in the master
branch with those from your feature
branch, use the following command after checking out to master
:
bash复制
1 | git merge -Xtheirs feature |
This command tells Git to prefer changes from the feature
branch during the merge.
2. Keeping Changes in master
Branch
If you want to keep changes from the master
branch and discard those from the feature
branch, use:
bash复制
1 | git merge -Xours feature |
This command tells Git to prefer changes from the master
branch.
Using These Strategies with Rebase
Rebasing is another common Git operation that can lead to conflicts. The -Xtheirs
and -Xours
options work slightly differently with git rebase
compared to git merge
.
1. Rebasing feature
onto master
and Keeping feature
Changes
If you’re on your feature
branch and want to rebase it onto master
while keeping your changes, use:
bash复制
1 | git rebase master -Xtheirs |
2. Rebasing feature
onto master
and Keeping master
Changes
If you want to prioritize changes from master
during the rebase, use:
bash复制
1 | git rebase master -Xours |
Understanding the Reverse Order in Rebase
The reverse behavior in rebasing can be confusing. When you rebase feature
onto master
, Git resets the feature
branch to the current master
commit and then applies your changes on top. In this context:
-Xtheirs
refers to thefeature
branch (since it’s the source of the changes).-Xours
refers to themaster
branch (since it’s the target).
For example, consider the following history:
复制
1 | E---F---G feature |
When you run git rebase master
, Git:
- Rolls back to the common ancestor commit (
A
). - Saves changes from
feature
temporarily. - Resets
feature
to the currentmaster
commit (C
). - Applies the saved changes (
E
,F
,G
) on top ofmaster
.
The final history looks like this:
复制
1 | A---B---C master |
Summary
To help you remember the strategies for resolving conflicts using -Xtheirs
and -Xours
, refer to the following table:
Current Branch | Command | Strategy | Outcome |
---|---|---|---|
master |
git merge feature -Xtheirs |
Keep feature changes |
Overrides master with feature changes |
master |
git merge feature -Xours |
Keep master changes |
Keeps master changes, discards feature changes |
feature |
git rebase master -Xtheirs |
Keep feature changes |
Overrides master with feature changes during rebase |
feature |
git rebase master -Xours |
Keep master changes |
Keeps master changes, discards feature changes during rebase |
Final Thoughts
Resolving Git conflicts efficiently is crucial for maintaining a smooth development workflow. By leveraging the -Xtheirs
and -Xours
options, you can quickly resolve conflicts without manually editing files. Understanding the nuances of these strategies, especially during rebasing, will help you manage your Git history more effectively.
Related Recommendations
- BugHunter
- How to Add a Folder Recursively to a Git Repository A Comprehensive Guide
- How to Fix the “Unable to Update Local Ref” Error in Git A Step-by-Step Guide
- How to Merge a Specific File from Another Branch in Git
- How to Remove a Submodule in Git A Step-by-Step Guide
- How To Abort A Rebase In Git
- How to fix “git pre-receive hook declined”?
- Why the Git Add Command Isn’t Working Troubleshooting Guide